Verify new users with Plivo
Eliminate fake accounts and verify customers— anywhere, in real time, with a 95% conversion rate.
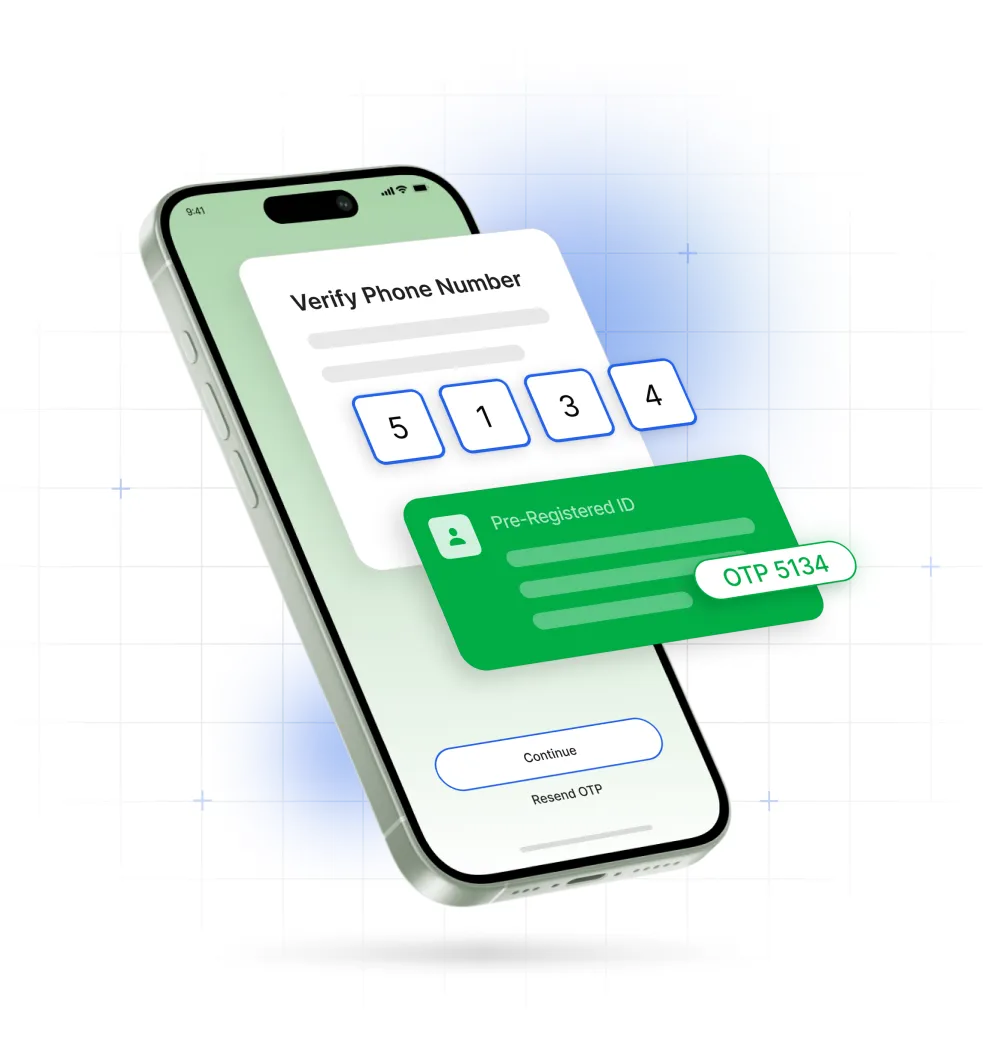
Thousands of large businesses globally use Plivo’s APIs
Plivo Verify is the best way to secure users & boost OTP conversions

Prevent SMS pumping from eroding your budget
Plivo Fraud Shield is an AI-driven model that automatically detects and blocks fraudulent messages. Set up your SMS Pumping Fraud Protection with a simple 1-click setup.

Plivo offers the lowest cost per verification
Zero charges for both Fraud Shield and OTP verification services.Only pay SMS, Voice, or WhatsApp charges
Plivo offers the lowest cost per verification
No hidden charges. Discover your potential savings with Plivo.
with Plivo
platforms for every x SMS sent
Only pay to verify REAL users
$0 Verification fee
$0 for Plivo Fraud Shield
Price Calculator


Verification Cost
Control Cost

Only pay to verify REAL users
$0 Verification fee
$0 for Plivo Fraud Shield
Plivo offers the lowest cost per verification
No hidden charges. Discover your potential savings with Plivo.
Zero charges for both Fraud Shield and OTP verification services
Only pay SMS, Voice or WhatsApp Charges
Make the Quick & Easy Transition to Plivo
Plivo’s Verify API is designed to ‘Go live in one sprint’. Our developer-first APIs and sample code can slash implementation time by 90% so your business never misses a beat!
1import sys
2sys.path.append("../plivo-python")
3
4import plivo
5
6client = plivo.RestClient('<auth_id>','<auth_token>')
7
8# Create Session (Send OTP)
9response = client.verify_session.create(recipient='<dest_number>')
10print(response)
11
12# Validate Session (Validate OTP)
13response = client.verify_session.validate(session_uuid='<session_uuid>', otp='<otp>')
14print(response)
1require "rubygems"
2require "/usr/src/app/lib/plivo.rb"
3include Plivo
4
5api = RestClient.new("<auth_id>", "<auth_token>")
6
7# Create Session (Send OTP)
8begin
9 response = api.verify_session.create(
10 nil,
11 "<dest_number>",
12 "",
13 nil,
14 nil,
15 nil
16 )
17 print response
18rescue PlivoRESTError => e
19 puts 'Exception: ' + e.message
20end
21
22# Validate Session (Validate OTP)
23begin
24 response = api.verify_session.validate(
25 "<session_uuid>",
26 "<otp>"
27 )
28 print response
29rescue PlivoRESTError => e
30 puts 'Exception: ' + e.message
31end
1let plivo = require('plivo')
2let client = new plivo.Client('<auth_id>','<auth_token>');
3
4// Create Session (Send OTP)
5client.verify_session.create({ recipient: '<dest_number>', method:'', channel:'', locale:'' }).then(function(response) { console.log(response) }).catch(function(error) {
6 console.error(error);
7 });
8
9// Validate Session (Validate OTP)
10client.verify_session.validate({id:'<session_uuid',otp:'<otp>'}).then(function(response) {console.log(response)}).catch(function (error) {
11 console.log(error)
12 });
1package main
2
3import (
4 "fmt"
5 "github.com/plivo/plivo-go"
6)
7func main() {
8 client, err := plivo.NewClient("<auth_id>", "<auth_token>", &plivo.ClientOptions{})
9 if err != nil {
10 fmt.Printf("Error:\n", err)
11 }
12 // Create Session (Send OTP)
13 responseTS, err := client.VerifySession.Create(plivo.SessionCreateParams{Recipient: "<dest_number>"})
14 if err != nil {
15 fmt.Print("Error", err.Error())
16 return
17 }
18 fmt.Printf("Response: \n%#v\n", responseTS)
19
20 // Validate Session (Validate OTP)
21 responseTG, err := client.VerifySession.Validate(plivo.SessionValidationParams{OTP: "<otp>"}, "<session_uuid>")
22 if err != nil {
23 fmt.Print("Error", err.Error())
24 return
25 }
26fmt.Printf("Response: \n%#v\n", responseTG)
27}
1<?php
2require '/usr/src/app/vendor/autoload.php';
3use Plivo\RestClient;
4
5
6// Create Session (Send OTP)
7try {
8 $response = $client->verifySessions->create("<dest_number>");
9 print_r($response);
10}
11catch (Exception $ex) {
12 print_r($ex);
13}
14
15// Validate Session (Validate OTP)
16try {
17 $response = $client->verifySessions->validate("<session_uuid>","<otp>");
18 print_r($response);
19}
20catch (Exception $ex) {
21 print_r($ex);
22}
23?>
1import java.io.IOException;
2import java.net.URL;
3import java.util.Collections;
4import com.plivo.api.Plivo;
5import com.plivo.api.exceptions.PlivoRestException;
6import com.plivo.api.models.verify_session.VerifySession;
7import com.plivo.api.models.verify_session.SessionCreateResponse;
8import com.plivo.api.models.message.Message;
9import com.plivo.api.exceptions.PlivoValidationException;
10import com.plivo.api.models.base.ListResponse;
11class Session {
12 public static void main(String[] args) {
13 Plivo.init("<auth_id", "<auth_token>");
14
15 // Create Session (Send OTP)
16 try {
17 SessionCreateResponse response = VerifySession.creator(
18 "",
19 "<dest_number>", "", "", "", "")
20 .create();
21 System.out.println(response);
22 }
23 catch (PlivoRestException | IOException e) {
24 e.printStackTrace();
25 }
26 // Validate Session (Validate OTP)
27try {
28 SessionCreateResponse response = VerifySession.validation("<session_uuid>","<otp>").create();
29 System.out.println(response);
30 }
31
32 catch (PlivoRestException | IOException e) {
33 e.printStackTrace();
34 }
35 }
36}
1using System;
2using System.Collections.Generic;
3using Plivo;
4using Plivo.Exception;
5namespace dotnet_sdk
6{
7 class Session
8 {
9 static void Main(string[] args)
10 {
11 var api = new PlivoApi("<auth_id>", "<auth_token>");
12 // Create Session (Send OTP)
13 try {
14 var response = api.VerifySession.Create(
15 recipient:"<dest_number>"
16 );
17 Console.WriteLine(response);
18 }
19
20 // Validate Session (Validate OTP)
21 try {
22 var response = api.VerifySession.Validate(
23 session_uuid: "<session_uuid>",
24 otp:"<otp>"
25 );
26 Console.WriteLine(response);
27 }
28
29 catch (PlivoRestException e){
30 Console.WriteLine("Exception: " + e.Message);
31 }
32}
33}
34}
1// Create Session
2curl 'https://api.plivo.com/v1/Account/<auth_id>/Verify/Session/' \
3--header 'Content-Type: application/json' \
4--header 'Authorization: Basic xxx' \
5--data '{
6"recipient": "<dest_number>",
7"channel":"sms",
8"url":"<callback_url>",
9"method":"POST",
10"app_uuid":"<app_uuid>"
11}'
12
13// Validate Session
14curl 'https://api.plivo.com/v1/Account/<auth_id>/Verify/Session/<session_uuid>/' \
15--header 'Content-Type: application/json' \
16--header 'Authorization: Basic xxx' \
17--data '{
18"otp":"<otp>"
19}'

Simplify compliance and go-live instantly
Bypass regulatory paperwork and go live instantly in countries like the US, India, and the UK using pre-registered sender IDs (e.g., PLVRFY, PLVSMS) and templates. Send OTPs globally in multiple languages.
Let’s find the right plan for your business
Customize Plivo’s OTP solution with ease

Seamlessly auto-fill OTPs on Android
When a user receives an OTP on their Android device, Plivo can configure the code to auto-fill into the app, eliminating the need for users to manually type in the OTP.
Configure, control and execute
Customize your OTP settings to send messages in multiple languages, switch templates, adjust configurations, and easily manage channels. No more complex code changes!
Plivo’s Key Differentiators
Plivo is a Trusted Partner for Superior Support, Guaranteed Delivery, and Simple Pricing
Secure cloud
communications
Frequently Asked Questions
What is the difference between verification & authentication?
Verification and authentication are typically used interchangeably, but they aren’t the same thing. Verification occurs at signup. It ensures that a user is who they say they are. Authentication occurs every time a user logs in. Plivo Verify can be used for both verification and authentication.
What’s the difference between SMS verification and voice verification?
Both are great options, but they have different benefits.
- SMS verification is fast and easy for users to complete.
- SMS verification has great reach: almost all mobile devices support SMS functionality.
- Voice verification provides an accessible alternative for individuals who may have visual disabilities.
- Voice verification works best for customers who only have access to a landline, as landlines don’t support SMS.
- Voice verification can be a reliable alternative or fallback in cases of delays or failures in SMS delivery. Voice is prioritized on carrier networks, resulting in higher delivery rates compared to SMS.
- Voice offers significantly richer data points for analytics, enabling users to gain deeper customer insights and optimize conversions.
Is 2FA the same as OTP for verification?
Two-factor authentication, or 2FA, refers to the use of two different types of authentication factors to verify a user's identity. These factors can come from any of the following three categories.
- Something you know: This could be a password, PIN, or the answer to a security question.
- Something you have: This could be a smartphone (to receive an SMS or use an authenticator app), a smart card, or a hardware token.
- Something you are: This refers to biometric data, like a fingerprint, facial recognition, or retina scans.
A one-time password (OTP) is valid for only one login session or transaction, and it relies on something you have. After entering a password (something you know), you might be sent an OTP via SMS to your phone (something you have), which you must then enter to gain access.
What is SMS verification?
SMS verification adds an extra layer of security by using two-factor authentication (2FA) to verify users’ identities. SMS verification helps ensure that the person trying to access the account or register for the service has a mobile device tied to that account. This can help prevent unauthorized access, even if someone gains access to the user's username and password.
How does SMS verification work?
Here are the steps in the SMS verification process:
- A user provides their mobile number to log in to an account or register for a service.
- The system then sends a request to Plivo to initiate the SMS verification process for that mobile number.
- Plivo generates a one-time password OTP) — a unique code that can be used for this one instance for verification.
- The OTP is sent via SMS to the user's mobile number. Plivo also keeps a copy of the OTP to check it against the user's input.
- The user receives the OTP in an SMS message on their phone and enters the OTP into the website or application to which they’re trying to log in or sign up.
- The user’s entry is is sent to Plivo. Plivo verifies whether it matches the OTP that was originally generated and sent to the user.
- If the OTPs match, Plivo verifies the user. If not, Plivo may resend the OTP,or the user may have to initiate the process again.
- Once the user is verified, they can proceed to log in to their account or complete their registration.
Articles about Verify API

Authentication vs. Authorization: What's the Difference?
In the interconnected world of apps, websites, and digital services, ensuring secure user access is more critical than ever. That’s where authentication and authorization come into play. These two terms often appear side-by-side in conversations about cybersecurity and user access, but they’re far from interchangeable.
Think of authentication as verifying your identity at the door, and authorization as the VIP list determining what areas you can access once inside. Both are essential for keeping digital spaces secure, but their roles are distinct—and understanding the difference is key to building safer systems and more seamless user experiences.
In this blog, we’ll break down the fundamentals of authentication and authorization, explore how they work together, and examine why they matter for individuals and organizations alike.
How does authentication verify user identity?
Authentication is the cornerstone of digital security, tasked with verifying that a user or entity is genuinely who they claim to be. Without authentication, systems cannot differentiate between legitimate users and malicious actors attempting unauthorized access.
At its core, authentication involves a user providing credentials—such as a username and password—that are compared against stored data. If the credentials match, the system permits access. However, traditional methods like passwords have vulnerabilities, prompting the adoption of more advanced techniques.
What are the common types of authentication methods?
Authentication mechanisms can be classified into three main categories based on the type of credentials required:
- Something you know: Includes passwords, PINs, and answers to security questions. These methods rely on the assumption that only the authorized user knows the required information.
- Something you have: Examples include physical devices like security tokens, mobile phones for OTP delivery, or smart cards. These add an extra layer of security by requiring possession of an item.
- Something you are: Biometric authentication leverages unique physical attributes like fingerprints, iris scans, or facial recognition, making it one of the most secure forms of identity verification.
Combining these methods through multi-factor authentication (MFA) strengthens security by requiring two or more forms of verification.
Why is multi-factor authentication (MFA) critical?
While traditional authentication methods offer a basic level of security, they can be vulnerable to attacks such as phishing or credential theft. This is where multi-factor authentication (MFA) comes in—by requiring two or more verification methods, MFA significantly reduces the risk of unauthorized access. For instance, a banking application might require both a password (something you know) and an OTP sent to your mobile device (something you have) before granting access.
MFA mitigates common vulnerabilities of single-factor authentication by making it harder for attackers to breach systems, even if one credential is compromised. It is particularly important for high-security environments such as financial institutions or cloud services.
How does authentication operate in cloud environments?
With businesses rapidly shifting to cloud-based platforms, authentication must evolve to meet the challenges of remote access and global connectivity. Cloud computing, with its shared infrastructure and global accessibility, demands robust authentication mechanisms. Traditional username-password combinations are often insufficient, so advanced approaches like token-based authentication and Single Sign-On (SSO) are widely used. These methods simplify access for users while maintaining strict security standards.
For example, SSO enables users to authenticate once and access multiple applications seamlessly. Coupled with standards like OAuth 2.0 and OpenID Connect, SSO ensures both security and convenience, making it an integral part of modern authentication in cloud environments.
How do authentication and authorization work together?
Authentication and authorization are integral processes that work in sequence to protect systems and data. Authentication identifies who the user is, while authorization determines their permissions within the system. Together, they ensure that only verified users gain access to the resources they are allowed to use, forming a robust framework for digital security.
Why must authentication always precede authorization?
Authentication and authorization are sequential processes that work in tandem to secure systems and data. Authentication verifies a user’s identity, forming the foundation for authorization to define what the user can do within the system. Without authentication, a system cannot determine whether a user is legitimate, making it impossible to assign permissions accurately.
For example, consider an enterprise resource management system. Authentication ensures a user, such as a department manager, is genuinely who they claim to be. Once authenticated, authorization evaluates their role and grants access to department-specific data while restricting other sensitive areas, such as payroll records for other departments.
What protocols effectively integrate authentication and authorization?
- OpenID Connect (OIDC)
OIDC, built on OAuth 2.0, focuses on user authentication by verifying identity and providing ID tokens to applications. It is particularly useful in Single Sign-On (SSO) environments, enabling users to authenticate once and access multiple applications seamlessly. - OAuth 2.0
OAuth 2.0 primarily handles authorization. It issues access tokens that grant limited permissions to third-party applications. For instance, a user can authorize a travel app to access their calendar to book flights without sharing their login credentials.
Together, OIDC and OAuth 2.0 provide a cohesive framework for managing authentication and authorization, ensuring secure and streamlined access control.
How do authentication and authorization complement each other in IAM systems?
Identity and Access Management (IAM) systems rely on the synergy between authentication and authorization to provide comprehensive security. While authentication confirms a user’s identity, authorization enforces granular access controls based on predefined policies.
For example:
- A marketing analyst authenticates into a shared cloud platform.
- Authorization allows access to customer analytics dashboards but restricts access to sensitive financial data meant for the finance team.
This integration not only enhances security but also improves the user experience by ensuring users can seamlessly access the resources they need without encountering unnecessary barriers.
What are the strengths and weaknesses of traditional authentication methods?
Traditional authentication methods often rely on verifying something a user knows, such as a password or PIN. While straightforward and familiar, these methods have inherent weaknesses:
- Password-based authentication:
- Strengths: Universally understood and simple to implement.
- Weaknesses: Susceptible to phishing, brute-force attacks, and credential stuffing. Users often reuse or create weak passwords, making them a common attack vector.
- Knowledge-based authentication (KBA):
- Strengths: Uses answers to security questions, adding an extra layer of protection.
- Weaknesses: Answers can often be guessed or researched, especially when questions rely on personal information.
These methods, while widely used, require additional safeguards to address their vulnerabilities.
How do biometric and possession-based methods enhance authentication?
Authentication methods based on something a user has or is, provide a higher level of security:
- Possession-based authentication:
Examples include physical devices like smart cards, security tokens, or mobile phones used to receive one-time passwords (OTPs).- Strengths: Tied directly to the user's possession, making them harder to replicate.
- Weaknesses: Devices can be lost or stolen, potentially compromising security.
- Biometric authentication:
Employs unique physical traits like fingerprints, retina scans, or voice recognition.- Strengths: Difficult to forge and highly reliable when implemented correctly.
- Weaknesses: Biometric data, if compromised, cannot be replaced, raising significant privacy concerns.
These methods often form the foundation of multi-factor authentication (MFA) systems, combining possession or biometric factors with traditional credentials to mitigate risks.
What are adaptive and passwordless authentication techniques?
Advanced authentication techniques are emerging to address the evolving threat landscape and user demands for convenience:
- Adaptive authentication:
Uses machine learning and context-aware policies to evaluate risk factors, such as location, device, or login time.- Example: A system might prompt for additional verification if a user logs in from an unusual location.
- Strengths: Dynamically adjusts security measures based on risk, improving both security and usability.
- Passwordless authentication:
Eliminates the reliance on traditional passwords, using methods like biometrics, hardware tokens, or magic links sent to a user’s email.- Strengths: Reduces phishing risks and enhances user convenience.
- Weaknesses: Requires advanced infrastructure and user education for widespread adoption.
These approaches represent the future of secure and user-friendly authentication systems.
What are the key differences and similarities between authentication and authorization?
Authentication and authorization serve distinct purposes in access control systems:
- Authentication: Focuses on verifying identity. It answers the question, "Who are you?" and allows only legitimate users to log in. Examples include passwords, biometric scans, or OTPs.
- Authorization: Determines what a user is allowed to do after they’ve been authenticated. It answers, "What are you allowed to access?" For instance, an authenticated user might be able to view files but not edit them.
The main distinction lies in their roles: authentication validates identity, while authorization defines permissions.
How do tokens facilitate both processes?
In modern access control systems, tokens play a critical role in separating authentication and authorization:
- ID Tokens:
- Issued during authentication to confirm a user’s identity.
- Typically contains user details such as name, email, and login time.
- Example: OpenID Connect generates ID tokens after a user logs in.
- Access Tokens:
- Issued during authorization to define the permissions granted to the user or application.
- Allow a user to interact with specific resources (e.g., files, APIs) without revealing sensitive credentials.
- Example: OAuth 2.0 uses access tokens to permit third-party apps to access user data within predefined limits.
By segregating authentication (ID tokens) and authorization (access tokens), systems maintain both security and clarity in managing access.
How do authentication and authorization complement each other?
Authentication and authorization are complementary processes, working together to provide robust access control:
- Authentication establishes trust: Ensures that only legitimate users enter the system.
- Authorization enforces boundaries: Restricts user actions based on predefined policies.
For example, in a corporate email system:
- Authentication verifies an employee’s identity via a company-issued login.
- Authorization determines whether the employee can access confidential documents or edit shared files.
Together, these processes create a multi-layered security approach, minimizing risks like unauthorized access and data breaches.
Why are both authentication and authorization critical for complete security?
Neither authentication nor authorization can independently secure a system. Relying solely on authentication might let verified users access sensitive areas they’re not permitted to view, while exclusive reliance on authorization without authentication would grant access without ensuring the user is legitimate.
For example:
- A cloud storage system might authenticate a user with valid credentials but use authorization to restrict access to sensitive financial reports, ensuring that only authorized roles, such as CFOs, can view them.
This synergy is particularly vital in regulatory compliance environments like HIPAA, where access to sensitive information is strictly governed.
Why is Plivo’s Verify API the ideal solution for user authentication?
Implementing secure and efficient authentication in today’s complex digital landscape requires solutions that are not only robust but also easy to integrate. This is where Plivo’s Verify API shines, offering a comprehensive toolset to streamline user verification while minimizing fraud risks and operational overhead.
How does Plivo simplify global user verification?
Plivo’s Verify API enables businesses to verify users in over 200+ countries effortlessly. Unlike traditional solutions that require navigating complex compliance hurdles, Plivo offers pre-registered sender IDs and pre-approved templates for regions like the US, UK, and India. This means you can go live instantly, without worrying about regulatory paperwork.
What makes Plivo’s authentication approach stand out?
- Multi-channel delivery for maximum reach:
Plivo supports OTP delivery across SMS, voice, and WhatsApp, ensuring reliable communication even in areas with inconsistent network connectivity. Upcoming support for RCS and email further expands its versatility. - High conversion rates:
With a 95% OTP conversion rate, Plivo delivers a seamless experience for end-users. Features like Android auto-fill ensure that OTPs are effortlessly entered, reducing user frustration and boosting engagement. - Customizable OTP settings:
Businesses can easily configure language preferences, templates, and delivery channels without requiring complex code changes. This flexibility allows organizations to tailor the authentication experience to their audience.
How does Plivo prevent fraud and reduce costs?
One of the standout features of Plivo’s Verify API is its ability to combat SMS pumping fraud—a common and costly issue for businesses relying on OTP-based authentication.
- AI-driven Fraud Shield:
Plivo’s Fraud Shield uses machine learning to detect and block fraudulent activity in real time, preventing financial losses caused by illegitimate OTP requests. The solution requires minimal setup, enabling fraud protection with a simple one-click configuration. - Cost-efficient verification:
Unlike many competitors, Plivo charges only for the communication channels used, with no hidden fees for verification itself. This ensures businesses maintain control over their costs without sacrificing security.
How does Plivo make integration effortless?
- Quick deployment:
Designed with developers in mind, Plivo’s Verify API offers comprehensive documentation, sample code, and SDKs that slash implementation time by 90%. Businesses can go live within a single sprint. - Developer-first approach:
Plivo provides 24/7 technical support through Slack and phone calls, ensuring that developers receive immediate assistance. The guaranteed same-day response time eliminates bottlenecks during critical phases of integration.
Don't let verification headaches slow you down—start using Plivo's reliable and scalable solution today! Get started now and unlock seamless authentication for your app.

Best practices for multi-factor authentication account recovery
Multi-Factor Authentication (MFA) is an essential safeguard for protecting sensitive information. However, as crucial as it is for security, the MFA recovery process can sometimes be a double-edged sword. If users lose access to their authentication method, they risk being locked out of their accounts. Therefore, a robust MFA recovery process should be a critical part of any authentication strategy.
Let’s walk through best practices for MFA account recovery to ensure your users can easily and securely regain access to their accounts.
Authentication requirements for account recovery
While traditional MFA methods provide excellent security, the MFA recovery process requires a slightly different approach. Recovery methods must be easily accessible, and memorable, and allow for a slower authentication process. Recovering an account isn't something most users would be required to do regularly.
The key requirements for a recovery system are:
- Long-term memorability or access: Users need to easily retrieve their recovery method, even if they don’t use it regularly.
- A slower authentication process is acceptable: Since account recovery is infrequent, a slight delay in authentication is fine as long as security is not compromised.
- Widely usable: The recovery method must be accessible and practical for most users across different devices and locations.
The right balance is essential. Your recovery process should be secure enough to prevent unauthorized access but user-friendly enough to prevent frustration.
Plivo’s Verify API, which supports multiple channels like SMS and in-app push notifications, can be an excellent tool for ensuring users have quick, secure access to their recovery methods.
What are the options for account recovery?
Gone are the days of relying on security questions for account recovery. The National Institute of Standards and Technology (NIST) has since recommended shifting away from these outdated methods due to their vulnerability. Today, the most reliable account recovery options involve using possession-based methods or account activity details.
1. Possession methods
Possession-based recovery methods are more secure than knowledge-based methods like security questions. Examples include:
- Backup codes: These are typically one-time-use codes that can be generated during the initial MFA setup. Users should store these codes securely in case of device loss or other issues. While they may seem simple, they offer a solid layer of security.
- Passkeys: A passwordless option that syncs private keys across devices, making it easier for users to recover their accounts without needing to remember complex passwords. Although passkeys are still being adopted, they offer a promising solution for both MFA and recovery.
Implementing these methods provides a secure fallback when users lose access to their primary authentication methods. Plivo’s Verify API can easily integrate into your system to deliver SMS-based recovery codes, offering both security and simplicity for users who need to regain access.
2. Account activity details
Another way to strengthen your recovery process is by leveraging account activity details. For example, asking users to confirm recent transactions or other identifiable actions can serve as a powerful recovery tool. These methods provide additional layers of security, helping to confirm a user's identity when primary credentials are unavailable.
How can social proof enhance account recovery processes?
Digital services and online platforms such as social networks or apps use trusted contacts or social proof to enhance their recovery processes. This could be a friend or family member who can verify the user’s identity. For example, platforms like Apple and Facebook use recovery contacts, allowing users to set up people who can help them regain access if needed.
However, this method works best for social networks with a large, established user base. If your service doesn’t have this feature built-in, focusing on other recovery options—such as backup codes and passkeys—can still provide strong security and ease of use.
How to strengthen your account recovery process?
To improve your account recovery process, consider the following recommendations:
- Register additional authentication methods: Ensure that users register multiple recovery methods during account setup. This gives them options to access their account if they lose access to one method.
- Design recovery processes based on data sensitivity: The higher the value of the data you're protecting, the more robust your recovery process should be. For sensitive services like financial applications, additional security layers are necessary.
- Require successful MFA setup before new methods: Before enabling new MFA methods, ensure users have successfully completed the MFA setup process to avoid issues during recovery.
- Prompt users about available recovery options: Regularly remind users of the backup methods available to them, particularly when logging in from new devices or after a password change.
Enhancing recovery process security
When enhancing your recovery process, keep these security measures in mind:
- Implement waiting periods: For sensitive recoveries, a waiting period can act as a deterrent for unauthorized access attempts. This gives you time to review and confirm that the recovery request is legitimate.
- Maintain MFA during recovery: Don’t deactivate MFA when users are trying to recover their accounts. This ensures that multiple authentication steps are still in place, preventing unauthorized access.
The MFA recovery process should always remain as secure as possible, even if it’s a bit slower than regular authentication. By adding layers of security, such as SMS or app-based MFA, you can ensure that both you and your users stay protected.
Simplify account recovery with Plivo’s Verify API
Plivo’s Verify API streamlines the MFA recovery process with secure, multi-channel options tailored to your business needs. By integrating Verify API into your authentication system, you can ensure users regain access efficiently while maintaining high-security standards.
Key features of Plivo’s Verify API:
- Multi-Channel support: Deliver recovery codes through SMS, voice, or in-app push notifications. With support for global reach across 220+ countries, Plivo ensures reliable account recovery even in regions with strict messaging regulations.
- Fraud prevention at no extra cost: Plivo’s built-in Fraud Shield detects and blocks fraudulent SMS activity, safeguarding your business from unnecessary costs and security breaches.
- Zero compliance hurdles: Pre-registered sender IDs and templates eliminate regulatory paperwork, allowing you to go live instantly in key markets like the US, UK, and India.
- Seamless integration: Plivo’s developer-first APIs and detailed documentation make it easy to integrate Verify API into your existing workflows. With sample code in popular languages like Python and Java, you can go live in one sprint.
- Scalability: Whether supporting a small user base or scaling to millions of users, Plivo’s infrastructure ensures consistent and reliable performance, even during peak traffic.
Why choose Plivo?
- Cost-Effective: Pay only for channel costs (SMS, voice, or WhatsApp) with no hidden fees or additional charges for verification or fraud prevention.
- Proven performance: Achieve a 95% OTP conversion rate across multiple channels, ensuring seamless user recovery experiences.
- Developer-Friendly: Cut implementation time by 90% with ready-to-use sample code and robust support from Plivo’s engineering team.
By leveraging Plivo’s Verify API, businesses can deliver a hassle-free, secure recovery experience while reducing support costs and protecting user data. Whether scaling globally or enhancing regional workflows, Plivo ensures your multi-factor authentication system remains intact during recovery, minimizing vulnerabilities and maximizing user satisfaction.
Take the next step with Plivo’s Verify API
Empower your business with a secure, cost-effective, and seamless account recovery solution. Whether you’re looking to improve OTP conversion rates, prevent fraud, or streamline user authentication, Plivo’s Verify API delivers the tools you need.
Get started today—integrate our Verify API in under a sprint and experience unparalleled reliability, global scalability, and expert support. Book a demo or request trial access now to see how Plivo can transform your account recovery process.

How to send a WhatsApp verification code
Sending a WhatsApp verification code may seem like a simple process, but many users face frustrating issues such as delayed codes, incorrect phone number formatting, or connectivity problems. These challenges can lead to delays in account setup or two-factor authentication (2FA), disrupting the user experience and compromising security.
In this guide, we’ll walk you through how to send a WhatsApp verification code seamlessly, troubleshoot common problems, and ensure your verification process is both efficient and secure.
What is a WhatsApp verification code?
A WhatsApp verification code is a time-sensitive, one-time password (OTP) sent to a user’s mobile phone to verify their identity during account setup or when accessing certain features. This code is generated by WhatsApp and sent via SMS or WhatsApp message.
Use cases for WhatsApp verification codes
Businesses
WhatsApp verification codes are widely used by businesses for user authentication, especially in industries like e-commerce, banking, and healthcare. For example:
- E-commerce: Retailers use WhatsApp verification codes to authenticate users during account signups or to verify a payment method.
- Healthcare: Medical services use WhatsApp for appointment bookings, sending verification codes to confirm patient identity before proceeding with bookings.
Personal use
Individual users may need WhatsApp verification codes when setting up a new account, transferring an account to a new phone, or when logging in from a new device.
Why you need a WhatsApp verification code
Security
The primary reason to use WhatsApp verification codes is to enhance security. As data breaches and identity theft become increasingly common, a simple password is no longer sufficient to secure an account. WhatsApp verification codes provide an additional layer of protection, ensuring only authorized users can access an account.
Account setup
Verification codes are essential when setting up new WhatsApp accounts. When a user installs WhatsApp for the first time or moves to a new phone, they must verify their phone number using a code sent to them via WhatsApp. This ensures that the number is linked to a legitimate account and is in the user’s possession.
Two-factor authentication
For businesses, implementing WhatsApp verification codes is a key component of two-factor authentication (2FA). This second step in the authentication process reduces the risk of unauthorized access by requiring the user to confirm their identity not only through their password but also through a verification code sent to their phone. This adds a crucial layer of security to sensitive operations like banking or personal account management.
How to send a WhatsApp verification code
Sending a WhatsApp verification code can be done in just a few simple steps, especially when using an API service like Twilio. Here's a guide to help you set up and send a WhatsApp verification code:
1. Create a Twilio account and get API keys
First, sign up for a Twilio account if you don’t have one. Once you’ve registered, go to the Twilio console, where you can generate the necessary API keys for integration. These keys are essential for sending messages programmatically through the Twilio platform.
2. Activate WhatsApp in Twilio console
Once you have your Twilio account set up, go to the Messaging section of the Twilio Console and select WhatsApp. You’ll need to activate WhatsApp in your account. This step involves using the Twilio Sandbox for WhatsApp, which allows you to test the service before going live. Follow the instructions in the console to link your phone number to the sandbox.
3. Get a Twilio WhatsApp-enabled number
After activating WhatsApp, you'll be assigned a WhatsApp-enabled number through Twilio. This number will be used to send WhatsApp messages, including your verification codes.
4. Write the code to generate a verification code
Next, write the logic to generate a one-time password (OTP) or verification code. This can be done with simple code that generates a random 6-digit number (or another format if needed). This OTP will be sent to the user via WhatsApp.
5. Send the WhatsApp message with the verification code
Using the Twilio API, send a WhatsApp message containing the verification code to the user’s phone number. The following code snippet in Python demonstrates how to send the verification code:

This message will contain the OTP, which the user will need to enter into your app or service to complete the verification process.
6. Test the WhatsApp verification code
Once you’ve set up the system, use Twilio’s testing tools to ensure the message is being sent properly. You can also test your WhatsApp messaging in the Twilio Sandbox before deploying it to a live environment.
7. Go live
After successful testing, you can switch from the sandbox to your production environment, making the WhatsApp verification system available to real users.
By following these steps, you’ll be able to integrate WhatsApp verification into your service and provide users with a seamless, secure verification process.
Troubleshooting issues with WhatsApp verification codes
Common problems:
- Code not received: This is one of the most common issues. Users may not receive the verification code due to network issues, incorrect phone number format, or phone number registration conflicts.
- Delayed messages: Sometimes, the WhatsApp verification code may be delayed due to server issues or high traffic on WhatsApp’s network.
- Code expiry: Users often fail to enter the verification code within the valid time window, causing the code to expire.
Solutions:
- Check phone number format: Always verify that the phone number includes the correct country code.
- Ensure stable network: Make sure that the user has a stable internet connection, as this is essential for receiving WhatsApp messages.
- Avoid overuse of same number: Ensure the number is not already registered with WhatsApp, which may prevent the code from being sent.
Best practices for sending WhatsApp verification codes
Timing
Make sure that the verification code is sent at the right time. Timing is crucial, especially when there’s a short window for users to enter the code before it expires. Always test delivery times to ensure that messages arrive promptly.
Security
Ensure that verification codes are transmitted securely to avoid any potential interception. Use secure channels and encryption to safeguard both the code and the phone number.
User Experience
The process of entering the verification code should be seamless. To improve the user experience, ensure that:
- Users receive clear instructions about what to do if they don’t receive the code.
- Provide a link for users to easily request a new code if necessary.
- Communicate the expiration time of the code, ensuring users know when to expect the next one.
How Plivo simplifies WhatsApp verification
Plivo not only simplifies the process of sending WhatsApp verification codes but also provides additional advantages that can improve the overall efficiency and security of your verification system.
With Plivo’s Verify API, you can easily verify new users in real-time, with a conversion rate of 95%. This ensures a seamless, fraud-free experience for your users, reducing the likelihood of fake account registrations. You can go live in over 150 countries within just minutes, using Plivo's pre-registered sender IDs and templates to bypass regulatory paperwork.
Here’s how Plivo’s solutions make it easier and better than other providers like Twilio:
- Alerts and Notifications: Plivo offers best-in-class conversion rates, ensuring that your messages are delivered reliably to users and enhancing authentication across multiple channels.
- Fraud Prevention: Plivo’s Fraud Shield solution helps protect your business by eliminating fake accounts and saving on SMS pumping fraud expenses at no extra cost.
- Cost Efficiency: With Plivo, there are no hidden charges for verification. You only pay for channel costs, ensuring significant savings on each verification attempt.
- Seamless Integration: Plivo’s developer-first approach allows for quick implementation. With sample code and a one-sprint setup, your business can be up and running in no time, with 90% less implementation time than other solutions.
- Customizable OTP Settings: Plivo lets you customize OTP settings, such as sending messages in multiple languages or easily switching templates and configurations, all without complex code changes.
- Auto-Fill OTP: When users receive an OTP on their Android devices, Plivo can configure the code to auto-fill into the app, eliminating the need for manual entry and improving the overall user experience.
- Quick Support: With Plivo, you get round-the-clock support via Slack and phone calls, ensuring you get same-day responses whenever you need assistance.
Plivo’s Verify API is designed to streamline user verification while saving you time and costs, making it the ideal solution for businesses looking to secure and scale their verification processes with WhatsApp.
Get started with Plivo today and optimize your verification process for better efficiency, security, and cost savings!
It’s easy to get started. Sign up for free.
Create your account and receive trial credits or get in touch with us