Migrating from Twilio to Plivo is a seamless and painless process. The two companies’ API structures, implementation mechanisms, XML structure, SMS message processing, and voice call processing are similar. We wrote this technical comparison between Twilio and Plivo APIs so that you can scope the code changes for a seamless migration.
Understanding the differences between Twilio and Plivo development
Most of the APIs and features that are available on Twilio are also available on Plivo and the implementation mechanism is easier as the steps involved are almost identical. This table gives a side-side comparison of the two companies’ features and APIs. An added advantage with Plivo is that not only can you code using the old familiar API/XML method, you can also implement your use cases using PHLO (Plivo High Level Objects), a visual workflow builder that lets you create workflows by dragging and dropping components onto a canvas — no coding required.
Plivo account creation
Start by signing up for a free trial account that you can use to experiment with and learn about our services. The free trial account comes with free credits, and you can add more as you go along. You can also add a phone number to your account to start testing the full range of our voice and SMS features. A page in our support portal walks you through the signup process.
You can also port your numbers from Twilio to Plivo, as we explain in this guide.
Migrating your voice application
As mentioned earlier, you can migrate your existing application from Twilio to Plivo by refactoring the code, or you can try our intuitive visual workflow builder PHLO. If you prefer the API approach, you can follow one of the voice quickstart guides based on your preferred language and web framework. Plivo offers server SDKs in seven languages: Python, Node.js, .NET, Java, Python, Ruby, and Go. For another alternative that lets you evaluate Plivo’s SMS APIs and their request and response structure, use our Postman collections.
How to make an outbound call
Let’s take a look at the process of refactoring the code to migrate your app from Twilio to Plivo to set up a simple Python application to make an outbound call by changing just a few lines of code.
Alternatively, you can implement the same functionality using one of our PHLO templates. For example, if you want to make an outbound call, your PHLO would be this:

How to receive an incoming call
You can migrate an application for receiving and handling an incoming call from Twilio to Plivo just as seamlessly, as in this example:
Here again, you can implement the same functionality using one of our PHLO templates. Your PHLO would look like:

For more information about migrating your Voice applications to Plivo, check out our detailed use case guides, available for all seven programming languages and PHLO.
How to forward an incoming call
You can migrate an application for forwarding an incoming call from Twilio to Plivo just as seamlessly, as in this example:
Here again, you can implement the same functionality using one of our PHLO templates. Your PHLO would look like:
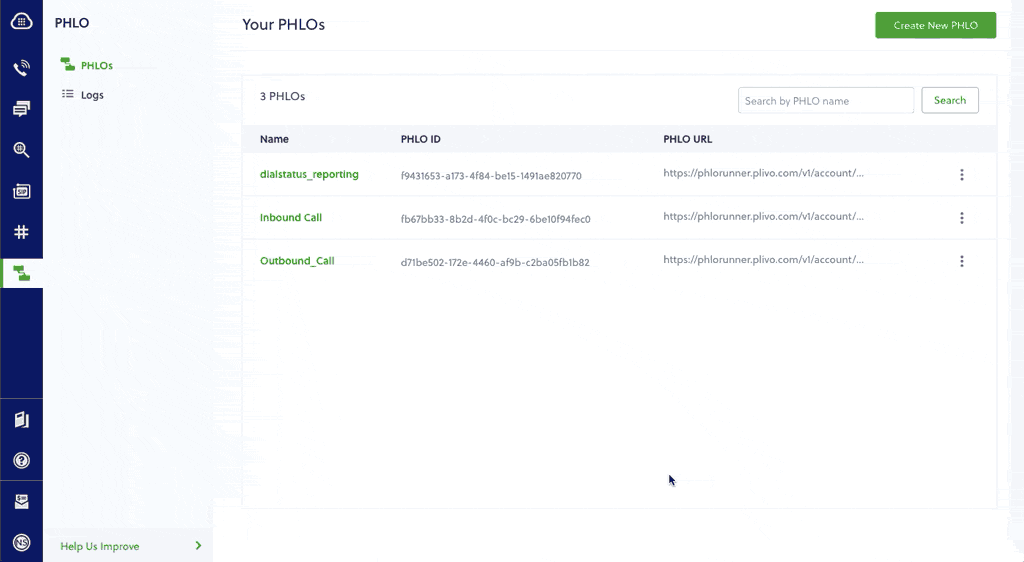
For more information about migrating your Voice applications to Plivo, check out our detailed use case guides, available for all seven programming languages and PHLO.
More use cases
You can migrate your applications serving other use cases too.
- IVR
- Voice-controlled virtual assistant
- Number masking
- Supervisor coaching
- PINless conference
- Conference with PIN
- Voicemail
- Voice alerts broadcasting
- Voice survey
- Dial status reporting
- Screen incoming calls
- Record a call
Simple and reliable
And that’s all there is to migrate your Python voice app from Twilio to Plivo. Our simple APIs work in tandem with our Premium Communications Network. See for yourself — sign up for a free trial account.