A virtual assistant can help your business if you have clients who call your phone number. Interactive voice response (IVR) helps you to automate call reception by routing callers to the most appropriate department or the agent most qualified to meet their needs. Among its many advantages, IVR can provide increased operational efficiency, a stronger brand image, and better customer insights.
A voice-controlled virtual assistant is one step ahead of the legacy Touch-Tone/DTMF controlled one because of the flexibility it allows end-users. They can just speak into their phone’s microphone to provide input to control the call.
Building a voice-controlled virtual assistant using Plivo’s automatic speech recognition (ASR) feature in Ruby using Ruby on Rails is simple. This guide shows you how to set up a voice-controlled IVR phone tree to a Plivo number and manage the call flow when the call reaches the Plivo voice platform. To see how to do this, we’ll build a rails application to receive an incoming call and use the GetInput XML element to capture speech input and implement a simple IVR phone system.
Prerequisites
Before you get started, you’ll need:
- A Plivo account — sign up for one for free if you don’t have one already.
- A voice-enabled Plivo phone number if you want to receive incoming calls. To search for and buy a number, go to Phone Numbers > Buy Numbers on the Plivo console.
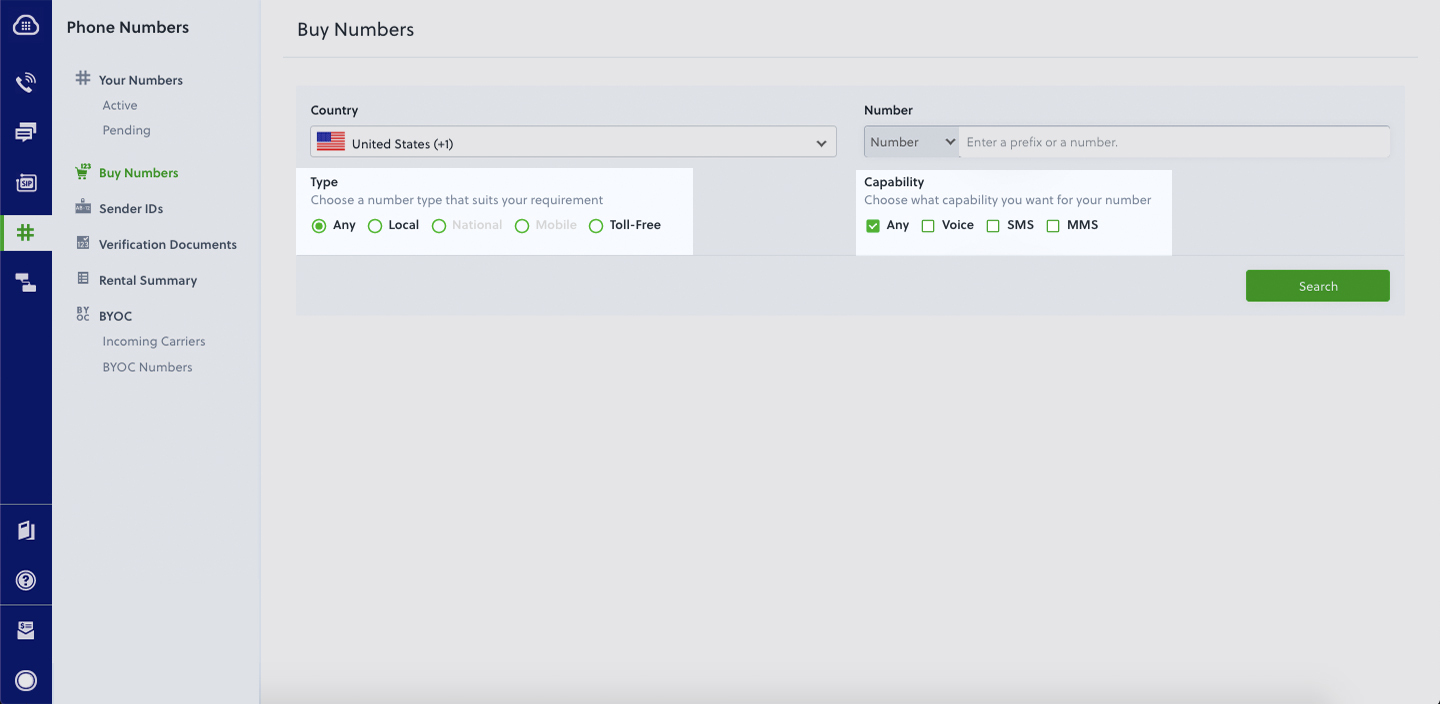
- Rails and Plivo Ruby packages.
- ngrok — a utility that exposes your local development server to the internet over secure tunnels.
How it works

Create a Rails application to create a voice-controlled virtual assistant
First, you need to install Rails if you haven’t installed it already. Use the command gem install rails, or use bundler or RVM to install it. Add a new Rails project with boilerplate code with the command rails new myrailsapp. This will create a myrailsapp directory with the necessary folders and files for development. Then add the Plivo Ruby gem (gem ‘plivo’, ‘~> 4.16.0’) as a dependency in the gemfile and use the command bundle install to install it.
Once you’ve installed Rails and the Plivo Ruby SDK, change to the newly created myrailsapp project directory and run rails generate controller Plivo voice to create a Rails controller to handle incoming calls on a Plivo number. To handle an incoming call, you need to return an XML document from the URL configured as the answer URL in the application assigned to the Plivo number. The Ruby SDK can manage the XML document generation, and you can use the GetInput XML element to capture speech inputs and implement a simple IVR phone system. Use this code in the PlivoController class in app/controllers/plivo_controller.rb file:
Test the code locally
Add a route for the inbound function in the PlivoController class. Open the config/routes.rb file and add this line after the inbound route: get ‘plivo/virtual_assistant’. To run the code on the rails server, use the command rails server. You should see your basic server application in action on http://127.0.0.1:3000/plivo/virtual_assistant/.
Expose the local server to the internet using ngrok
Once you see the application working locally, the next step is to connect the application to the internet to return the XML document to process the incoming call. For that, we recommend using ngrok, which exposes local servers behind NATs and firewalls to the public internet over secure tunnels.
Install ngrok, but before you start the ngrok service, whitelist ngrok by adding it to the config.hosts list in the config/environments/development.rb file with this command. You’ll face a Blocked host error if you fail to add it.
Now run ngrok on the command line, specifying the port that hosts the application on which you want to receive messages (3000 in this case, as our local Rails application runs there):
Ngrok will display a forwarding link that you can use as a webhook to access your local server over the public network.

Test the link by opening the ngrok URL(https://e3b9-49-206-112-65.ngrok.io/plivo/virtual_assistant/) in a browser or HTTPie to check the XML response from the ngrok URL.

Connect the Rails application to a Plivo number
The final step is to configure the application as a Plivo voice application and assign it to a Plivo number on which you want to activate the voice-controlled virtual assistant.
Go to the Plivo console and navigate to Voice > Applications > XML, then click on the Add New Application button in the upper right.
Provide a friendly name for the application — we used “App-Virtual-Assistant” — and configure the ngrok URL https://e3b9-49-206-112-65.ngrok.io/plivo/virtual_assistant/ as the Answer URL. Select the HTTP verb as POST, then click Create Application.
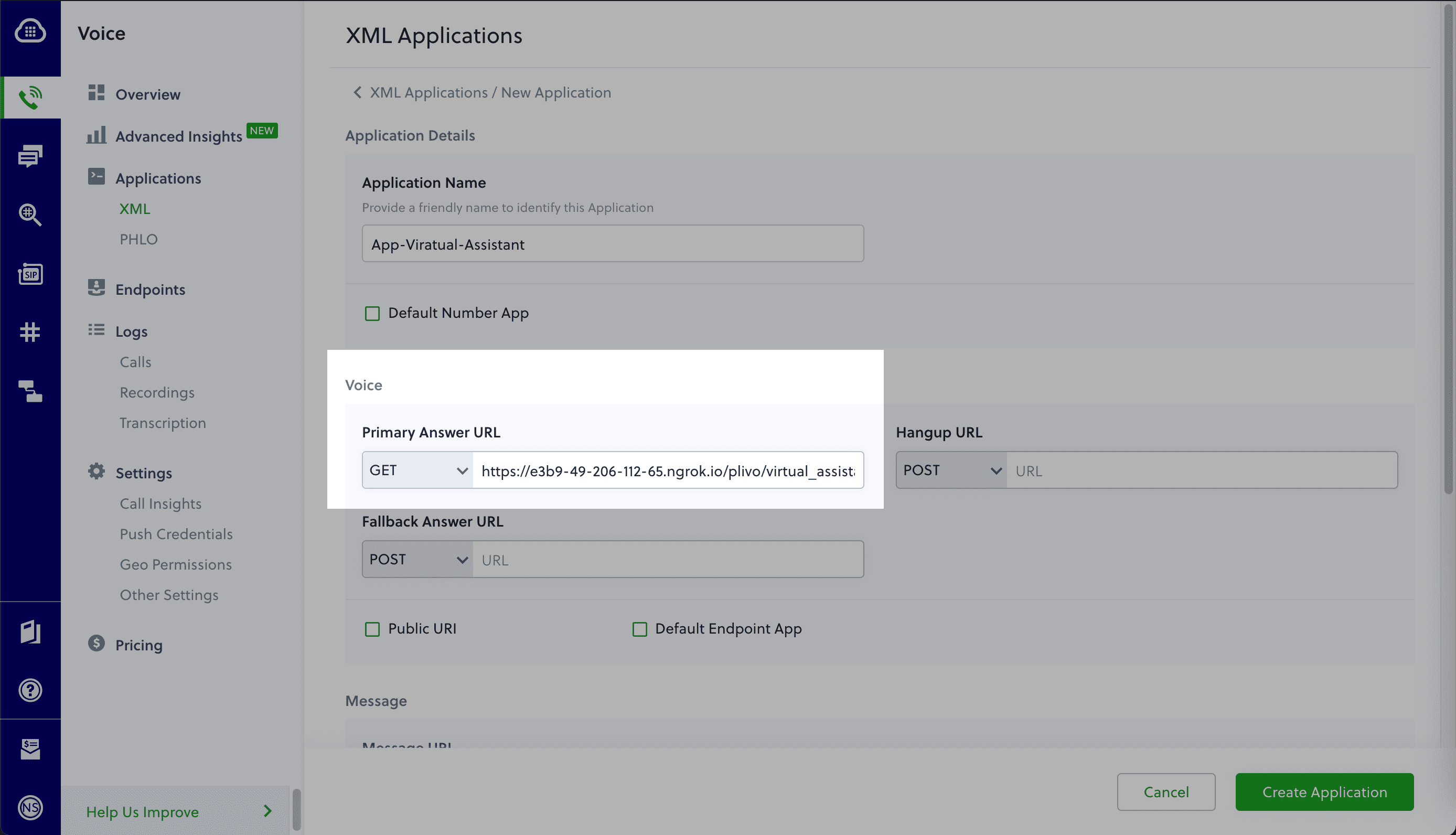
Now go to Phone Numbers > Your Numbers and click on the number to which you want to assign the application. From the Plivo Application drop-down, choose the voice application you just created. Finally, click Update Number.
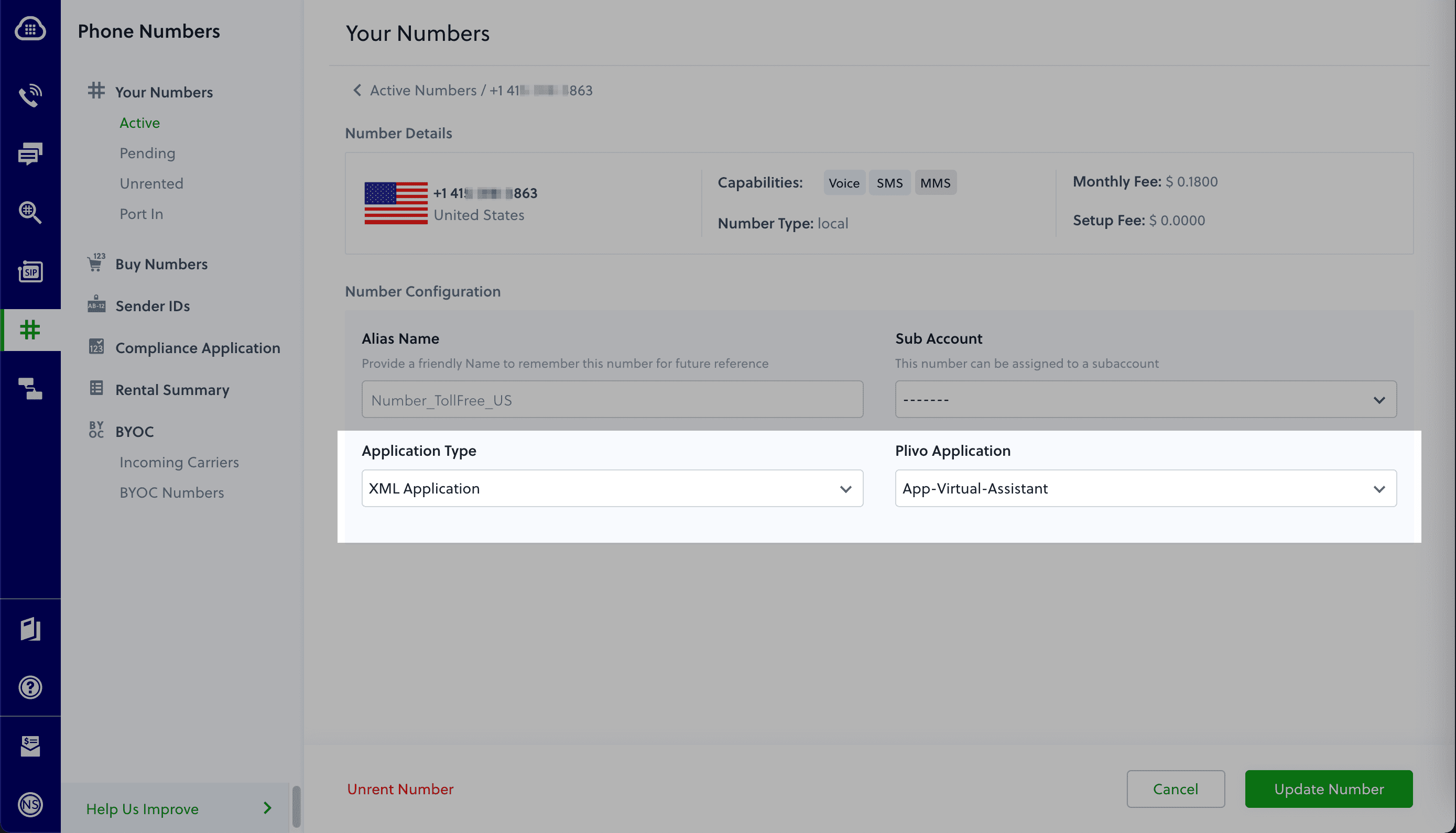
Test the application
Make a phone call to the Plivo number you selected. You should see that the VirtualAssistant rails application automatically routes the call to the Sales and Support departments based on the speech inputs received on the call.
And that’s how simple it is to set up a voice-controlled virtual assistant on a Plivo number and handle it using XML documents using Plivo’s Plivo’s Ruby SDK and a rails application. You can implement other use cases on the Plivo Voice platform, such as phone system IVR, call forwarding, and number masking, as your business requires.
Haven’t tried Plivo yet? Getting started is easy and only takes five minutes. Sign up today.